Data Structures | Types, Classification, and Applications
Published: 8 Sep 2024
Classification of Data Structure
Did you know that there are two primary categories of data structures, primitive and non-primitive, and that each is essential to programming? Ever wondered why your code becomes slow or hard to manage even when your logic seems fine? Many beginners struggle to choose the right data structure because they don’t know how each type works or where to use them. From arrays to trees, understanding the classification of data structures can make your programs faster, cleaner, and easier to build.
Classification of Data Structure in Computer Science |
---|
Linear Data StructureThe components of a linear data structure are arranged in a straight line, one after the other. With this form of organization, it is easy to browse through the data in a series because each element is related to the things that come before and after it. Linear data structures are simple to understand and use when the order of data matters. ExamplesThe most widely used forms of linear data structures are arrays, linked lists, queues, and stacks. Static Data StructureA static data structure is fixed in size, meaning that once it is created, you cannot change how much data it can hold. ExampleThis is an array, where you decide how many elements (or items) it will store, and that size remains the same throughout the program. Static structures are simple and efficient when you know exactly how much data you’ll be working with. Dynamic Data StructureA dynamic data structure can grow or shrink as needed. This means you don’t need to decide its size in advance. ExampleA linked list can keep adding new elements without running out of space, and you can also remove elements easily. Dynamic structures are flexible and work well when the amount of data changes frequently. Non-Linear Data StructureA non-linear data structure organizes data in a way that isn’t arranged straight. Instead of being placed one after another, the data is connected in multiple directions, allowing more complex relationships between elements. This structure is useful when you need to represent hierarchies, networks, or other complex connections where order doesn’t strictly matter. ExamplesGraphs and trees are the two most common forms of non-linear data structures. |
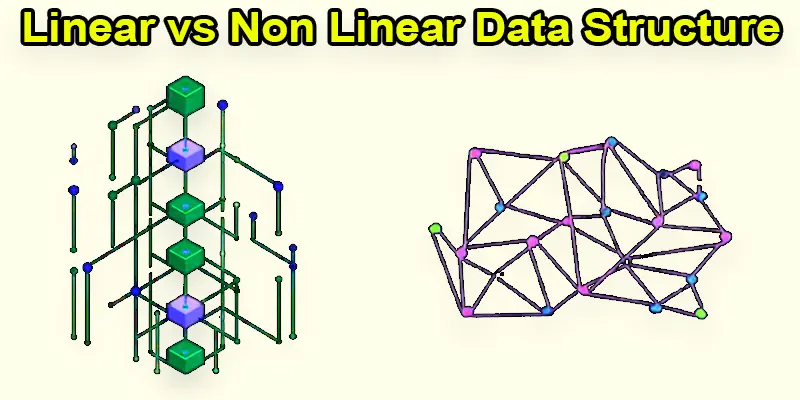
Why Data Structures are Needed in Programming
Data structures are needed to manage information efficiently.
- Faster data access: Quickly find or retrieve information, saving time in programs.
- Efficient data storage: Store data wisely, using memory efficiently and avoiding waste.
- Better problem-solving: Solve problems like sorting and searching more easily with a proper structure.
- Improved performance: Make programs run faster and handle data more smoothly.
Key Characteristics of Data Structures
Data structures have key characteristics that make them essential. They arrange information so that it is simple to locate, retrieve, and use. They also affect how quickly operations like searching and sorting are performed.
- Organization: How data is arranged and managed. Good data structures organize data in a way that makes it easy to find and use.
- Efficiency: How quickly and effectively data operations are performed. Efficient data structures help perform tasks like searching or sorting faster.
- Flexibility: How well the data structure adapts to changes. Some data structures can grow or shrink as needed, while others have a fixed size.
- Access Methods: The ways you can retrieve or modify data. Different data structures provide different methods for accessing and changing the data they store.
- Storage: How data is stored in memory. Data structures vary in how they use memory, with some being more space-efficient than others.
Major Advantages of Using Data Structures |
---|
|
Disadvantages or Limitations of Data Structures |
---|
|
How Data Structure Differs From Data Type in Programming
We’ve already covered data structures, but people often mix up data types and data structures. Let’s look at some key differences to help clarify things.
Data Structure | Data Type |
---|---|
Organizes and stores multiple values in a specific way (e.g., arrays, linked lists). | Defines a single type of value (e.g., integer, string). |
Manages and organizes collections of data efficiently. | Specifies what kind of data you are dealing with and how to use it. |
More complex, as it involves multiple values and organizational rules. | Simpler, focusing on single values. |
Manages memory for multiple values, often resizing or reallocating as needed. | Allocates fixed memory for one value based on its type. |
Supports advanced operations like searching, sorting, and linking data. | Supports basic operations like arithmetic or concatenation on single values. |
- Insertion: Adding new data to the structure, like putting a new book on a shelf.
- Deletion: Removing data from the structure, like taking a book off the shelf.
- Searching: Looking for a specific piece of data, like finding a book by its title.
- Traversal: Going through each item in the data structure one by one, like walking down a row of books and checking each one.
- Sorting: Arranging data in a specific order, like organizing books from A to Z.
- Merging: Combining two data structures into one, like merging two lists of books into a single list.
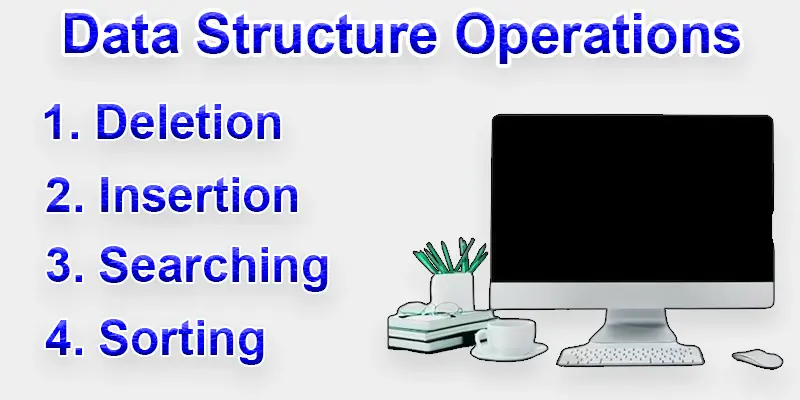
Basic Terminology Used in Data Structures
- Element: A single piece of data, like one item in a list or one number in a group.
- Node: A point in a data structure (like a tree or linked list) that holds data and can connect to other nodes.
- Array: A group of data components is stored in a fixed order, like a row of boxes where each box holds one item.
- Index: The position of an element in a data structure, like a specific seat number in a row of chairs.
- Pointer: A reference that points to the location of another element or node in a data structure, like a map showing where something is located.
Should you choose Data Science as a Career Path?
Yes, I’ve thought about choosing data science as a career. It’s an interesting field where you work with data to find useful insights and solve real-world problems. It also has great job opportunities and the chance to keep learning new things.
Real-Life Applications of Data Structures in Software
Data structures are essential tools in computer science that help organize data efficiently. They are used in many areas to make tasks like searching, sorting, and storing data faster and more effectively. Applications of data structures can be seen in things we use daily, like databases, operating systems, and even web browsers. By using the right data structure, we can solve complex problems more easily and improve the performance of software and systems.
- Databases: Data structures like hash tables and trees are used to store and organize large amounts of information in databases, making it quick and easy to search, update, or retrieve data.
- Operating Systems: Data structures like queues and stacks help manage tasks in an operating system, like which program runs next or how the computer remembers what to do next.
- Web Browsers: Browsers use stacks to manage the back and forward buttons, allowing you to return to the previous webpage easily.
- Social Networks: Data structures like graphs help represent connections between people on social media, showing who is connected to whom.
- Navigation Systems: GPS and navigation systems also employ graphs to determine the fastest or shortest path between two sites.
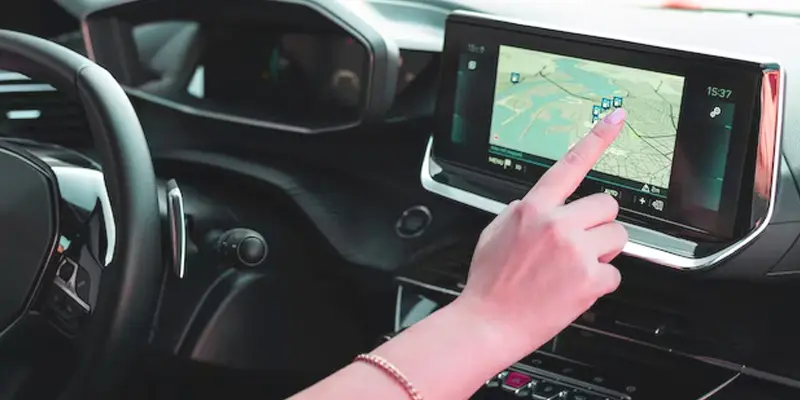
What is the Goal of Learning Data Structures
Data structures aim to help manage data in a way that makes it easy to use. They aim to make tasks like storing, finding, and modifying data quicker and more efficient. By choosing the right data structure, you can solve problems more effectively and improve the performance of programs.
Conclusion About Data Structure Learning
Understanding the classification and application of data structures is vital to solving problems efficiently in computer science. Different data structures are suited for various tasks, from organizing data in databases to managing tasks in operating systems. Selecting the proper data structure for each task can improve performance and make your programs run more smoothly. Classifying and applying these structures helps you handle data effectively and easily tackle complex challenges. Data structures aim to help manage data in a way that makes it easy to use.
FAQS – Data Structure
Data structures are helpful because they help organize data efficiently. They make it easier to store, find, and use information quickly, which improves the performance of programs and helps solve problems more effectively.
Learning about data structures is important because it helps you understand how to organize and manipulate data in the best way. This knowledge allows you to write more efficient code, which is essential for developing effective software and solving complex problems.
Primitive data structures are used in computer languages to hold basic data types like letters or numbers. Combinations of primitive types, such as arrays, lists, and trees, create more complicated non-primitive data structures. Primitive structures are simple, while non-primitive ones help manage larger, more complex data sets.
The four main types are Array, Stack, Queue, and Linked List. These help in storing and organizing data in different ways.
Data can be classified by type, structure, purpose, and usage. Each method helps in understanding how the data is stored and processed.
The main basis is similarity in characteristics or behavior. For example, data types are classified by the kind of value they store.
Classification means sorting items into categories. It is based on shared features or rules.
The two main classifications are the SI (International System) and the Imperial system. These systems define how we measure things like length, mass, and time.
The two major systems are Natural Classification (based on traits or structure) and Artificial Classification (based on use or function). Both help in different ways depending on the purpose.
The SI system uses standard units like the meter, kilogram, and second. It is simple, worldwide, and based on powers of 10.
The SI (Metric) system and the Imperial system are the most common. SI is used in most countries, while Imperial is mainly used in the USA.
SI is easy to use and convert. It’s used worldwide, which makes global communication and science easier.
It allows scientists to study, compare, and understand living and non-living things better. Without classification, science would be confusing.
Arranging books by subject or sorting clothes by type is classification. It helps us stay organized.
Yes, you can convert between SI and Imperial units. For example, 1 inch equals 2.54 centimeters.
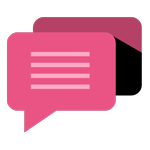
- Be Respectful
- Stay Relevant
- Stay Positive
- True Feedback
- Encourage Discussion
- Avoid Spamming
- No Fake News
- Don't Copy-Paste
- No Personal Attacks
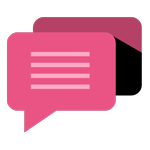
- Be Respectful
- Stay Relevant
- Stay Positive
- True Feedback
- Encourage Discussion
- Avoid Spamming
- No Fake News
- Don't Copy-Paste
- No Personal Attacks