Data Types Explained: Complete Guide from Beginner to Expert
Published: 14 Jan 2025
What is a Data Type?
A data type is a category that defines the kind of data a variable can hold in a program, such as numbers, text, or dates. It teaches the computer how to handle and store various values, including text, numbers, and true/false statements. The actions that can be performed on the data and the amount of memory required are specified by each data type. Strings (text), booleans (true or false), floats (decimal numbers), and integers (whole numbers) are examples of common data types. Understanding data types is important for writing programs that work correctly and efficiently.
10 Essential Data Types You Should Know
A data type is a way to categorize the data stored in a computer program. It informs the program about the kind of values that can be stored in a variable, including text, integers, and true/false values. Here are common data types include:
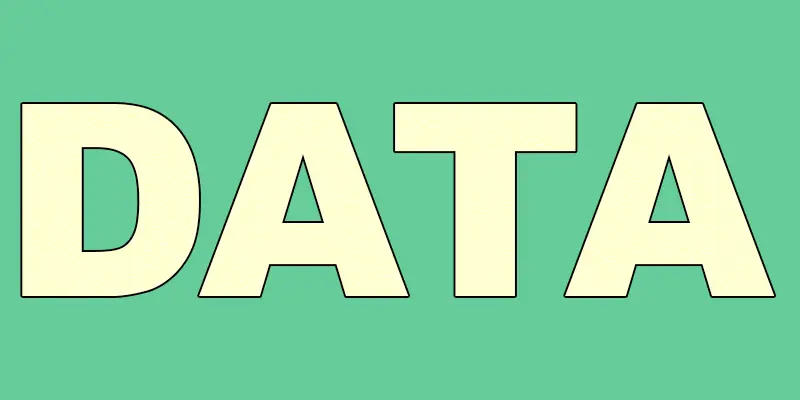
Integer
All integers, both positive and negative, as well as zero, are stored in an integer data type. Integers do not have decimal places or fractions, making them ideal for counting, measuring, and performing basic arithmetic operations like addition or subtraction. In most programming languages, integers are used when you need to work with values that don’t require a decimal, such as the number of items in a list or the result of a calculation. The integer data type is one of the most commonly used data types in programming.
Example of Integer
Here are some examples of integers:
- 10 (positive whole number)
- -3 (negative whole number)
- 0 (neither positive nor negative)
- 25 (positive whole number)
Float
A float data type is used to store numbers that have decimal points. It allows for more precision when working with values that are not whole numbers, like measurements or calculations involving fractions. Floats are useful when you need to represent values like weight, height, or temperature. They can also handle very large or very small numbers.
Example of Float
Here are a few examples of floats:
- 3.14 (a number with decimals)
- -0.75 (a negative number with decimals)
- 100.5 (a number with a decimal point)
String
A string data type stores text, such as words, sentences, or even a combination of letters and numbers. It can include letters, numbers, and special characters, but it’s always treated as text. Strings are useful when you need to work with names, addresses, or any other type of written information. They are usually enclosed in quotation marks to distinguish them from other data types.
Example of String
- “Hello” (a word)
- “1234” (numbers as text)
- “apple pie” (a combination of words)
Boolean
Values that can only be true or false are stored in boolean data types. Programming frequently uses it to make judgments, such as determining whether a condition is met. Boolean values help control the flow of a program, such as deciding whether to perform an action or not based on certain conditions. This type of data is essential for creating logical comparisons and controlling program behavior.
Example of Boolean
Here are a few examples of booleans:
- true (a statement that is correct)
- false (a statement that is incorrect)
Character (Char)
A character (char) data type stores a single letter, number, or symbol. It can hold any single character, like ‘a, ‘B,’ or ‘9’, and is typically enclosed in single quotation marks. Characters are useful when you need to work with individual letters or symbols in a program, such as processing text one character at a time. This data type is often used in string manipulation or in handling keyboard inputs.
Example of Character
- ‘a’ (a single letter)
- ‘7’ (a single number)
- ‘#’ (a symbol)
Array
A data type called an array is used to hold a group of objects of the same kind in a certain sequence. It allows you to group multiple values together, like a list of numbers or names, and access them using an index. Arrays are useful when you need to work with a set of related data, such as storing the scores of multiple students or the names of different cities. Each item in an array can be accessed quickly by its position, known as an index.
Example of Array
- [1, 2, 3, 4] (an array of numbers)
- [“apple,” “banana,” “cherry”] (an array of words)
- [10, 20, 30, 40, 50] (an array of integers)
Object
An object is a data type used to store a collection of related information, often in the form of key-value pairs. Each object can have different properties, and each property has a value. Objects are useful for grouping different kinds of data together, like storing information about a person, such as their name, age, and address. They assist in arranging data so that it is simpler to handle and deal with.
Example of Object
Here’s an example of an object:
- { “name”: “John”, “age”: 25, “city”: “New York” }
Null
The absence of any value or object is represented by the null data type. It is used when a variable or object hasn’t been assigned any data yet. In programming, null helps indicate that a value is intentionally empty or missing, and it’s different from zero or an empty string. It’s useful for checking if a variable has been properly initialized or if data is unavailable.
Example of Null
Here’s an example of null:
- Null (represents no value assigned)
In this case, the variable doesn’t hold any data. It is used to show that the value is empty or missing, not zero or an empty string.
Date
The date data type is used to store specific dates and times, such as a day, month, year, or even a full timestamp. It helps keep track of events or records when something happens. With the date data type, programs can easily handle and manipulate dates for tasks like scheduling, logging events, or calculating time differences. This data type ensures that the program understands and works with time-related information correctly.
Example of Date
Here’s an example of a date:
- “2024-11-20” (represents a specific date: November 20, 2024)
In this case, the date data type stores the exact date and can be used in programs to manage or track time-related information.
Double
The double data type is similar to a float, but it provides more precision for storing numbers with decimals. It can handle very large or very small numbers and is often used when you need to work with values that require more accuracy, such as scientific calculations or financial data. Doubles use more memory than floats, allowing them to store more digits after the decimal point. This makes them ideal for situations where precision is important.
Example of Double
Here are a few examples of doubles:
- 3.1415926535 (a precise value of pi)
- -0.000123456 (a very small negative number)
- 150.987654 (a number with more decimal places)
Importance of Data Types
Data types are important because they help the computer understand how to handle and store different kinds of data. When we define a data type, it ensures that the data is used correctly in calculations or comparisons. For example, you wouldn’t want to add text like “apple” to a number like 5, as it doesn’t make sense.
By using the right data type, programs can run faster and avoid errors. Data types also make sure the program uses memory efficiently, storing only what’s necessary. Without data types, programs would be confusing and harder to manage.
Conclusion about Data Types
Data types are essential in programming because they define what kind of data a variable can hold. They help the program understand how to store, use, and manipulate information correctly. By choosing the right data type, we can ensure that our programs run efficiently, avoid errors, and make the best use of memory. Whether it’s numbers, text, or dates, data types help organize and manage different kinds of data in a program.
FAQS – Data and Data Types
What are the different types of data types?
Data types are categories that specify the type of data a variable can hold. The main types of data include:
- Integer: Whole numbers (e.g., 5, -10)
- Float: Decimal numbers (such as 3.14 and -2.5) that float
- String: A string is a collection of characters or text, such as “Hello” or “1234.”
- Boolean: A value that can only be true or false is called a boolean.
- Character: One letter or symbol, such as “a” or “Z.”
- Array: A collection of similar data types stored together
- Object: A collection of related data (key-value pairs)
- Null: Represents an empty value
- Date: Used to store dates and times
What are the data types in PostgreSQL?
In PostgreSQL, the common data types include:
- Integer types: Such as integer (whole numbers), bigint (large integers), and smallint (smaller integers)
- Floating-point types: Such as real and double precision for numbers with decimals
- Character types: Such as char, varchar (variable-length strings), and text for storing text data
- Boolean: To store true or false values
- Date and time types: Such as date, timestamp, and time for storing date and time data
- UUID: For storing unique identifiers
- Array types: For storing a list of values of the same type
- JSON: For storing JSON data
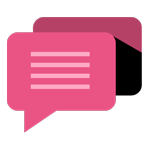
- Be Respectful
- Stay Relevant
- Stay Positive
- True Feedback
- Encourage Discussion
- Avoid Spamming
- No Fake News
- Don't Copy-Paste
- No Personal Attacks
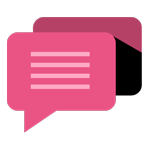
- Be Respectful
- Stay Relevant
- Stay Positive
- True Feedback
- Encourage Discussion
- Avoid Spamming
- No Fake News
- Don't Copy-Paste
- No Personal Attacks